Ex)
java
열기Note) 실행 결과
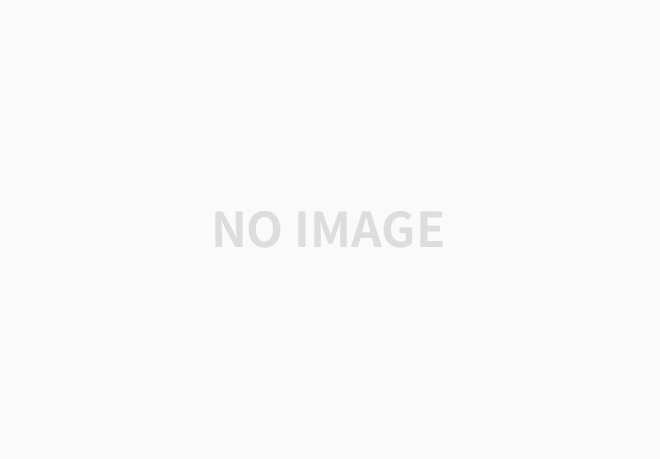
- pop(), peek(), empty(), full(), push()를 구현한다.
- stack의 특성에 따라서 배열에 저장된 값 중 가장 위의 값을 먼저 출력(FIFO)하는 구조로 만든다.
'자료구조와 알고리듬 With Java > [Study] BAEKJOON 프로그래머스 CodeUp LeetCode' 카테고리의 다른 글
Code up 3117 0은 빼! (0) | 2022.03.16 |
---|---|
Code up 2016 천단위 구분기호 (0) | 2022.03.16 |
Code up 1928 재귀함수 우박수 문제 (basic) (0) | 2022.03.16 |
Code up 1920 재귀함수를 이용한 2진수 변환 (0) | 2022.03.16 |
Code up 1714 숫자 거꾸로 출력하기 (0) | 2022.03.13 |